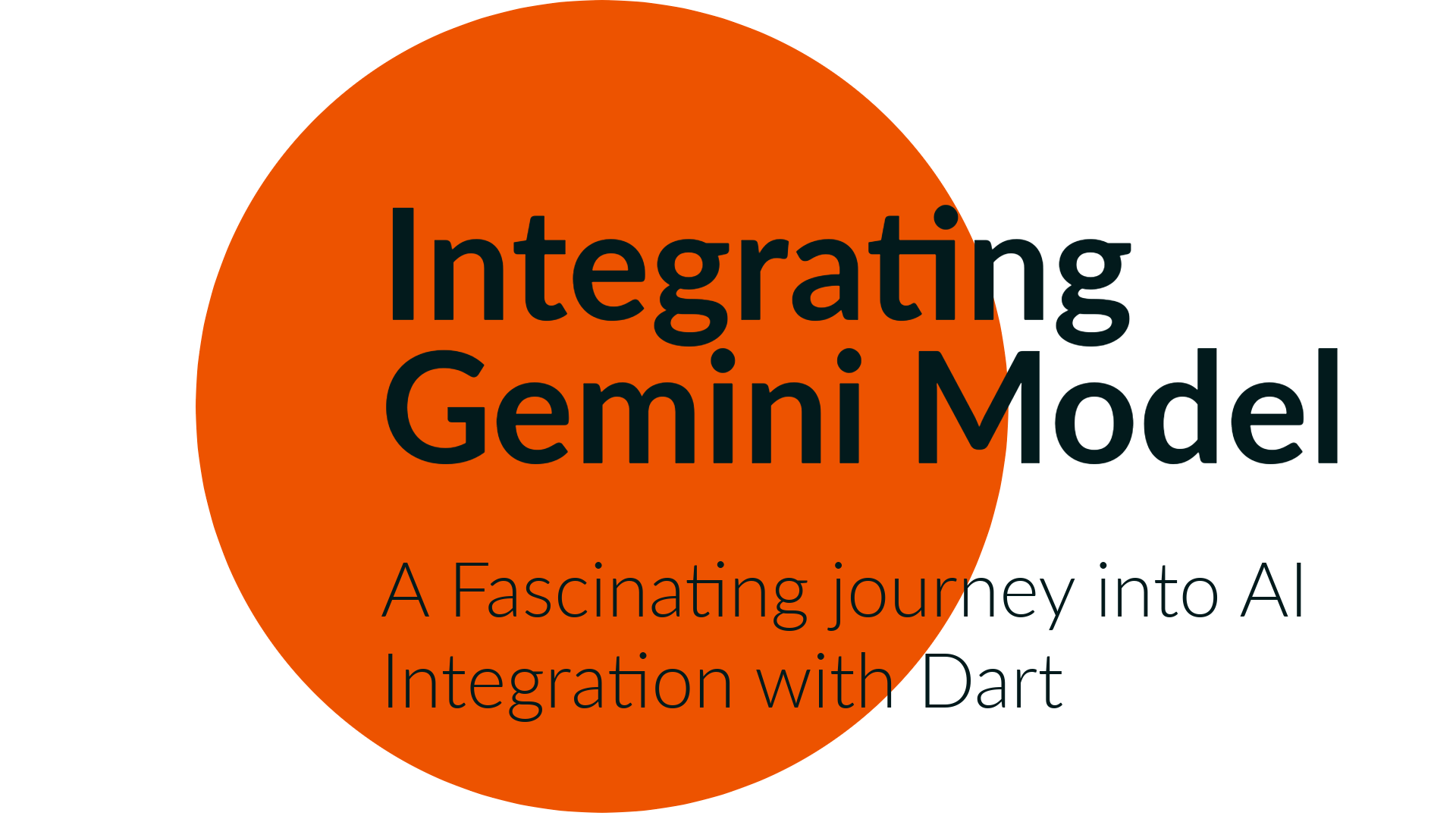
Linkedin Instagram Facebook X-twitter In today’s rapidly evolving tech landscape, the fusion of artificial intelligence (AI) and programming languages opens…
In today’s rapidly evolving tech landscape, the fusion of artificial intelligence (AI) and programming languages opens up new vistas of possibilities. Dart, known for its efficiency and versatility, stands at the forefront of this revolution. Combining Dart with powerful AI models like Gemini propels us into realms where creativity and innovation thrive.
In this blog post, we embark on a journey to integrate the Gemini model, a cutting-edge AI model developed by Google, with Dart, Google’s open-source, client-optimized programming language. We’ll delve into a Proof of Concept (POC) that illustrates how seamlessly Dart can harness the power of Gemini to generate content, spark creativity, and enhance user experiences.
Let’s dissect the Dart code snippet that orchestrates the integration with the Gemini model:
The initializeGenerativeModel
function initializes the Gemini model with specified configuration settings, including maximum output tokens, temperature, top-p, and top-k values. Additionally, it sets safety settings to ensure that the generated content adheres to community guidelines and filters out harmful material.
import 'package:google_generative_ai/google_generative_ai.dart';
GenerativeModel initializeGenerativeModel(String apiKey) {
// Configuration settings for content generation
final generationConfig = GenerationConfig(
maxOutputTokens: 4096,
temperature: 0.5,
topP: 0.9,
topK: 10,
);
// Safety settings to filter out harmful content
final safetySettings = [
SafetySetting(HarmCategory.harassment, HarmBlockThreshold.high),
SafetySetting(HarmCategory.hateSpeech, HarmBlockThreshold.high),
SafetySetting(HarmCategory.sexuallyExplicit, HarmBlockThreshold.high),
SafetySetting(HarmCategory.hateSpeech, HarmBlockThreshold.high),
SafetySetting(HarmCategory.dangerousContent, HarmBlockThreshold.high),
];
// Initializing the Gemini model
final generativeModel = GenerativeModel(
model: 'gemini-1.0-pro',
apiKey: apiKey,
generationConfig: generationConfig,
safetySettings: safetySettings,
);
return generativeModel;
}
The generateWordCount
function dynamically generates word counts based on the difficulty level selected by the user. It adjusts the distribution of easy, hard, and difficult words accordingly, ensuring a balanced and challenging experience.
Map<String, dynamic> generateWordCounts(int level) {
var difficultyDistribution = {
'easy': 0.8,
'medium': 0.15,
'hard': 0.05,
};
if (level > 1) {
final previousMediumCount = difficultyDistribution['medium']!;
final previousHardCount = difficultyDistribution['hard']!;
var totalAdjustment = 0.0;
difficultyDistribution['medium'] = difficultyDistribution['medium']! +
min(0.05 * level, 1 - difficultyDistribution['medium']! - previousMediumCount);
totalAdjustment += difficultyDistribution['medium']! - previousMediumCount;
if (level % 2 == 1) {
difficultyDistribution['hard'] = difficultyDistribution['hard']! +
min(0.05, 1 - difficultyDistribution['hard']! - previousHardCount);
totalAdjustment += difficultyDistribution['hard']! - previousHardCount;
}
if (totalAdjustment > 0) {
difficultyDistribution['easy'] = difficultyDistribution['easy']! - totalAdjustment;
}
}
final totalWords = 6 + (level - 1) * 2;
final wordLength = 7 + (level - 1);
final wordCounts = {
for (final difficulty in difficultyDistribution.keys)
difficulty: (totalWords * difficultyDistribution[difficulty]!).toInt(),
};
int remainingWords =
totalWords - wordCounts.values.fold(0, (sum, value) => sum + value);
if (remainingWords > 0) {
wordCounts['easy'] = wordCounts['easy']! + remainingWords;
}
return {
'wordCounts': wordCounts,
'totalWords': totalWords,
'wordLength': wordLength,
};
}
ThegenerateWordsByCategory
function leverages the Gemini model to generate words based on the selected category, difficulty level, and word length criteria. It constructs prompts tailored to the user’s preferences and retrieves word suggestions from the Gemini model.
Future<List<String>> generateWordsByCategory({
required dynamic model,
required String category,
required int numWords,
required int numberOfEasyWords,
required int numberOfHardWords,
required int numberOfDifficultWords,
required int wordLength,
}) async {
String prompt;
if (numberOfEasyWords > 1) {
if (numberOfHardWords > 1) {
if (numberOfDifficultWords > 1) {
prompt = 'Get $numWords words in the $category category. $numberOfEasyWords easy, $numberOfHardWords hard, $numberOfDifficultWords difficult. Word length <= $wordLength.';
} else if (numberOfDifficultWords >= 1) {
prompt = 'Get $numWords words in the $category category. $numberOfEasyWords easy, $numberOfHardWords hard, $numberOfDifficultWords difficult. Word length <= $wordLength.';
} else {
prompt = 'Get $numWords words in the $category category. $numberOfEasyWords easy, $numberOfHardWords hard. Word length <= $wordLength.';
}
} else if (numberOfHardWords >= 1) {
prompt = 'Get $numWords words in the $category category. $numberOfEasyWords easy, $numberOfHardWords hard. Word length <= $wordLength.';
} else {
prompt = 'Get $numWords words in the $category category. $numberOfEasyWords easy. Word length <= $wordLength.';
}
} else {
prompt = 'Get $numWords words in the $category category. Word length <= $wordLength.';
}
final content = [Content.text(prompt)];
final response = await model.generateContent(content);
final words = response.text.split('\n').map((line) => line.split(' ')[1]).toList();
return words;
}
The generateHints
function solicits hints for a given word from the Gemini model, maintaining the suspense and challenge while providing valuable clues to the user.
Future<List<String>> generateHints(String word, int numberOfHints, dynamic model) async {
final prompt = 'Give me $numberOfHints hints to guess the word "$word" without revealing the word itself.';
final content = [Content.text(prompt)];
final response = await model.generateContent(content);
final hints = response.text.split('\n')
.map((line) => line.trim().split('.'))
.where((hint) => hint.length > 1)
.map((hint) => hint[1].trim())
.toList();
return hints;
}
The main function serves as the entry point, orchestrating user inputs, category selection, level determination, word generation, and hint retrieval. It encapsulates the core logic of the integration, offering a cohesive and interactive user experience.
void main() async {
// Initialize the generative model
dynamic generativeModel = initializeGenerativeModel("YOUR_API_KEY");
final categories = ["animals", "body parts", "objects", "countries", "flowers", "fruits", "cities"];
// Display available categories to the user
print("Available categories:");
categories.asMap().forEach((index, category) {
print("${index + 1}. $category");
});
// Prompt user to select a category
print("Select a category (1-${categories.length}): ");
final int categoryIndex = int.parse(stdin.readLineSync()!) - 1;
final String selectedCategory = categories[categoryIndex];
// Prompt user to select a difficulty level
print("Select a difficulty level (1-7): ");
final int selectedLevel = int.parse(stdin.readLineSync()!) - 1;
// Generate word counts based on the selected difficulty level
Map<String, dynamic> wordCounts = generateWordCounts(selectedLevel);
int totalWords = wordCounts['totalWords'];
int wordLength = wordCounts['wordLength'];
int easyWords = wordCounts['wordCounts']['easy'];
int hardWords = wordCounts['wordCounts']['hard']!;
int difficultWords = wordCounts['wordCounts']['difficult'];
// Generate words based on the selected category and counts
List<String> words = await generateWordsByCategory(
model: generativeModel,
category: selectedCategory,
numWords: totalWords,
numberOfEasyWords: easyWords,
numberOfHardWords: hardWords,
numberOfDifficultWords: difficultWords,
wordLength: wordLength,
);
// Display generated words to the user
print("Words:");
for (int i = 0; i < words.length; i++) {
if (words[i].length <= wordLength) {
print("${i + 1}. ${words[i]}");
} else {
print("Error!");
print("${i + 1}. ${words[i]}"); // Print the word even if it exceeds length for clarity
}
}
// Prompt user to select a word index to get hints
print("Select a word index to get hints for (1-${words.length}): ");
final int wordIndex = int.parse(stdin.readLineSync()!) - 1;
// Generate hints for the selected word
List<String> hints = await generateHints(words[wordIndex], 3, generativeModel);
// Display hints to the user
print("Hints:");
for (final hint in hints) {
print("- $hint");
}
}
In this blog post, we embarked on a captivating exploration of integrating the Gemini model with Dart, unlocking a plethora of possibilities for content generation, gamification, and user engagement. By harnessing the power of AI within the Dart ecosystem, developers can create immersive experiences that captivate and inspire audiences worldwide.
Stay tuned for more insights, tutorials, and innovations at the intersection of AI and programming languages. Together, let’s push the boundaries of creativity and technology, shaping a future where the possibilities are limitless.
For more information on Dart and Gemini, explore the following resources:
Feel free to experiment with the code snippet provided and embark on your own journey of AI integration with Dart. The possibilities await!
Stay inspired, stay innovative, and happy coding!
As Tech Co-Founder at Yugensys, I’m passionate about fostering innovation and propelling technological progress. By harnessing the power of cutting-edge solutions, I lead our team in delivering transformative IT services and Outsourced Product Development. My expertise lies in leveraging technology to empower businesses and ensure their success within the dynamic digital landscape.
Looking to augment your software engineering team with a team dedicated to impactful solutions and continuous advancement, feel free to connect with me. Yugensys can be your trusted partner in navigating the ever-evolving technological landscape.
Linkedin Instagram Facebook X-twitter In today’s rapidly evolving tech landscape, the fusion of artificial intelligence (AI) and programming languages opens…
Linkedin Instagram Facebook X-twitter In this Blog Introduction The Journey Begins Seamless Integration The Moment Of Truth Embracing The Future…
Linkedin Instagram Facebook X-twitter OpenAI has just unveiled its comprehensive guide to writing prompts, offering five new strategies to harness the…
Linkedin Instagram Facebook X-twitter In this Blog Challenge Possibility Thinking Build a Resilient Mindset Surround Yourself with Positivity Set Realistic…